Authentication
To keep data on Attentive’s platform safe and secure, all apps connecting with Attentive’s APIs must authenticate when making API requests. This article describes the different methods of authenticating and authorizing apps with Attentive’s platform.
Types of authentication
Different types of apps require different authentication or authorization methods:
- Private apps use basic HTTP authentication.
- Public apps use OAuth 2.0.
HTTP authentication
Private applications must authenticate through basic HTTP authentication by using their API key as the Bearer Token when making calls to Attentive’s APIs. To generate a unique API key, you must create an app in the Attentive platform. You should treat your API key as a password. If it is accidentally shared, other users may be able to send messages to customers on your behalf. Use the /me endpoint to verify that you are authenticating correctly.
For a GraphQL API example:
curl --location --request POST 'https://api.attentivemobile.com/v1/graphql' \
--header 'Authorization: Bearer <Unique API Key>' \
--header 'Content-Type: application/json' \
--data-raw '{"query":"\nquery {\n viewer {\n installedApplication {\n installerCompany {\n id\n name\n }\n }\n }\n}\n\n","variables":{}}'
For a REST API example:
curl 'https://api.attentivemobile.com/v1/me' \
-X GET \
-H 'Authorization: Bearer <Unique API Key>' \
-H 'Content-Type: application/json' \
Note: If you are unable to access the Attentive platform, please send an email to api-tokens@attentivemobile.com for access.
OAuth 2.0
Public applications must use the OAuth 2.0 protocol. After an application is authorized, end users approve your app for access to their Attentive data and features, or to have your app fetch data from Attentive. This allows users to authorize your application to make requests without sharing any passwords.
Attentive’s APIs use OAuth 2.0 with the authorization code grant flow to issue access tokens on behalf of users.
Requirements
Before proceeding with this OAuth 2.0 section, you must meet the following expectations:
- You must create an application in the Attentive platform.
- Understand which APIs your application can access.
- Understand the scopes for each endpoint. See Authorization scopes for details.
Obtain Authorization
Making authorized requests to the Attentive platform requires that you are granted permission to access data.
In accordance with RFC-6749, three parties are involved in the authorization process:
- Server: the Attentive server
- Client: your application
- Resource: the end user data and controls
Authorization Flow
Attentive provides an authorization code to allow users to install your app. This authorization code must be exchanged for an access token, which does not expire. Since the token exchange involves sending your secret key, we recommend performing this on a secure location. For example, we recommend using a backend service, and not from a client such as a browser or from a mobile app.
For further information about this flow, see RFC-6749.
The following diagram and corresponding description illustrates the OAuth flow:
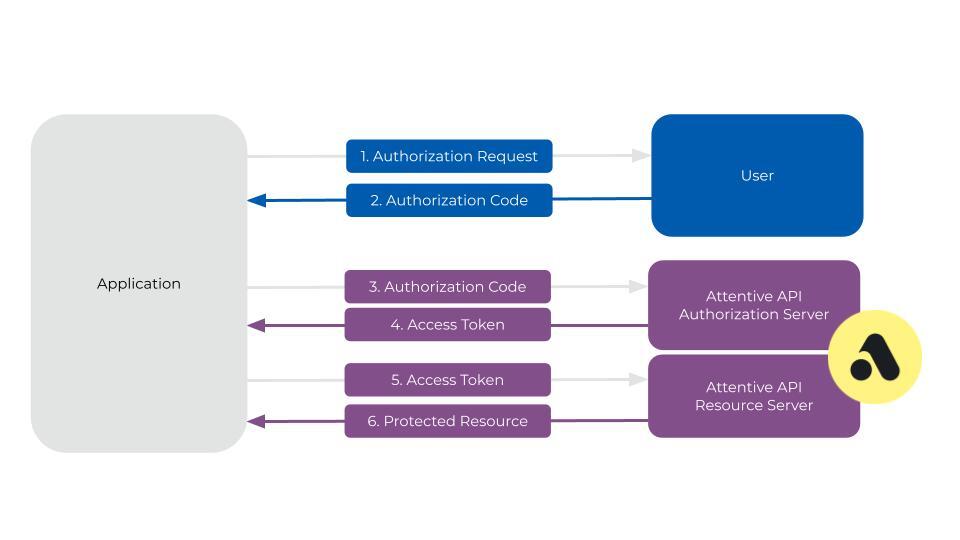
- The user makes a request to install the app.
- The app redirects to the distribution URL in Attentive to load the OAuth authorization page. The URL will be in the form of the following:
https://ui.attentivemobile.com/integrations/oauth-install?client_id={your clientid}&redirect_uri={the redirect uri}&scope={scopes}
Note the following: Attentive generates a distribution URL for each application automatically and it can be found on the Manage Distribution page. Theredirect url
must be one that is associated with the application and the same URL must be used in the call to get an access token. The scopes in the distribution URL must match the scopes that the app requires. The app can also optionally send along astate
parameter to be returned. It is strongly recommended to addstate
since this provides protection against attacks such as cross-site request forgery. See RFC-6749. - Attentive ensures the user is logged in, and then prompts the user to authorize the app.
-
The user agrees to the scopes and is redirected to the
redirect url
defined in the app settings. An authorizationcode
andstate
are passed back to the application as well added on to the redirect URL.https://exampleUrl.com/callback?code=NApCCg..BkWtQ&state=xyzABC123
- The app uses the temporary authorization code to send an access token request to Attentive’s Access Token endpoint. The authorization code will expire after two minutes if it is not used.
- Attentive returns an access token. The application must correlate each access token to each company that installs the app.
The app can now use this access token to make requests to Attentive's APIs on behalf of the company using the app.
Authorization Scopes
Scopes enable your application to access specific API endpoints on behalf of a user. The set of scopes you pass in your call determines the access permissions that the user is required to grant. In order to create Attentive applications, you need to understand the different scopes. Scopes provide Attentive users using third-party apps the confidence that only the information they choose to share will be shared, and nothing more.
Scopes
The following table outlines the various scopes for each corresponding endpoint.
Scope | Description | Required endpoint |
events:write
|
Read and write permissions to the Events API. | Custom Events |
ecommerce:write
|
Read and write permissions to the Ecommerce API. | Ecommerce |
subscriptions:write
|
Read and write permissions to the Subscribers API. | Subscribers |
attributes:write
|
Read and write permissions to the Attributes API. | Custom Attributes |
privacy_requests:write
|
Read and write permissions to the Privacy API. | Privacy Request |